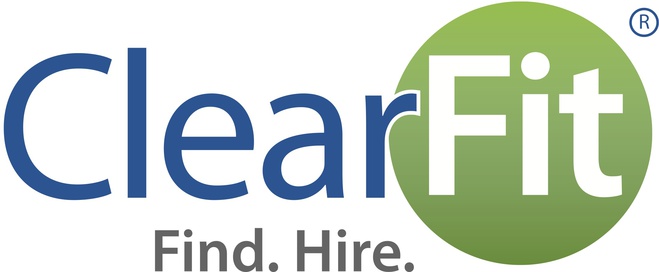
I am always doing that which I can not do, in order that I may learn how to do it.
Everything in Ruby is an object
1.is_a?(Object) # true Array.is_a?(Object) # true Object.is_a?(Object) # true
Specialized functions for various types of data
Used to store a list of things
a = Array.new # [] a.push(1) # [1] a.unshift('a') # ['a',1] a.insert(1, "index") # ['a', 'index', 1]
Useful when the order is important (queue)
a = [1,2,3,4] a.length # 4 a.pop # 1 a.length # 3 a.pop # 2 ...
Can be treated as a set
a = [9,3,4,1,2,3,4] a.uniq! # [9,3,4,1,2] a.sort! # [1,2,3,4,9]
Used to store characters
a = "hello" # "hello" a += " " # "hello " a << "world" # "hello world" a.concat(33) # "hello world!"
They are really an array of characters
a = "abcd" a[0..2] # "abc"
Nice functions to format text
a = "Aloha" a.downcase # "aloha" a.upcase # "ALOHA" a.capitalize # "Aloha"
Used as a key / value store
a = Hash.new # {} a[1] = "one" # { 1 => "one" } a["two"] = 2 # { 1 => "one", "two" => 2 } a.keys # [1, "two"] a.values # ["one", 2]
Useful when you want to perform a lookup
lookup = { "matt": "647-123-4444" } lookup[:matt] # "647-123-4444" lookup.fetch(:ben, "No number found")
A way of making your own objects
Grouping functions by purpose / category
class ClearFit def self.pitch "Find the right jobs for people" end end ClearFit.pitch
Knowing is not enough, you must apply; willing is not enough, you must do.